We had a user who was using a custom text field with the HTML5 validation as a sort of invite code system. The issue was that bots can easily bypass HTML5 validation, so some server-side validation is also needed. This is a short tutorial on how to implement custom server side validation for a custom field.
First, Add a Custom Text Field
Go to UsersWP > Form Builder > Add a text field.
Make sure you note the “Key” in this case “invite_code”.
Here, I have not added any HTML5 validation as it is not required if we are doing server-side validation.
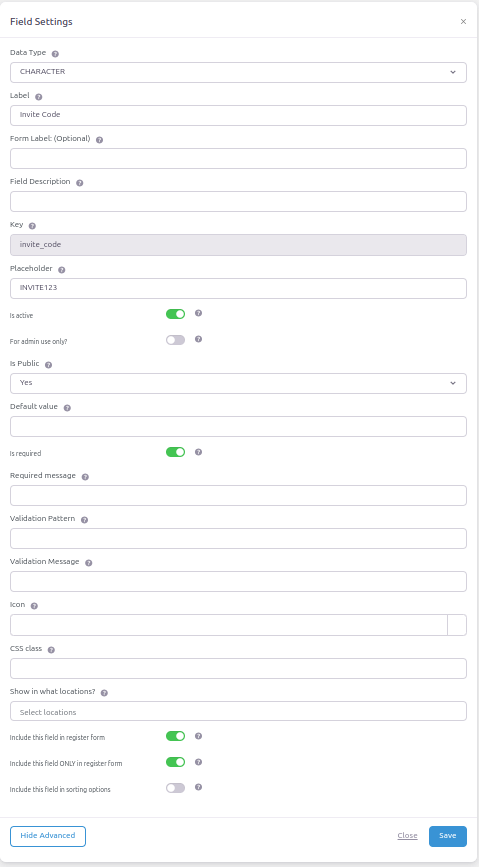
Add the field to the register form
Above, I have set this to “Include this field ONLY in the register form”.
Go to UsersWP > Form Builder > Register > Add the new field to the register form and drag it where you want it to show, I have added it as the first field.
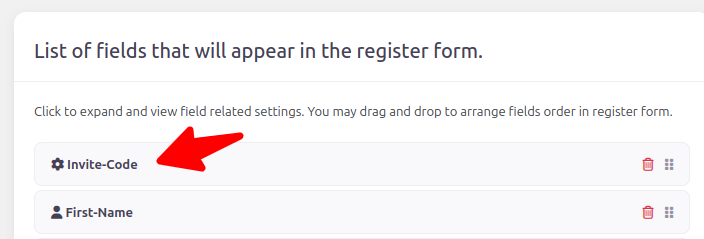
Add the server-side validation
Below we add a code snippet to validate the invite code, note that the “invite_code” key used has to match the field Key. We have a list of valid invite codes, this list can be as long or short as needed.
add_filter('uwp_validate_fields_before','_my_invite_code_check',10,3);
function _my_invite_code_check( $errors, $data, $type ){
// List of valid codes
$valid_codes = array(
'SAMPLE123',
'CAT345',
'BOB99',
'123456',
'POOL'
);
// only run chcks if we have data and its a register call
if (!empty($data) && $type === 'register') {
// if empty add an error
if(empty($data['invite_code'])){
$errors->add('not_logged_in', __('<strong>Error</strong>: Invite Code Empty', 'userswp'));
}elseif(!in_array($data['invite_code'],$valid_codes)){
// if used code is not in our list then add an error
$errors->add('not_logged_in', __('<strong>Error</strong>: Invite Code Not Valid', 'userswp'));
}
}
return $errors;
}
Result
Users will only be able to register if they have a valid invite code.
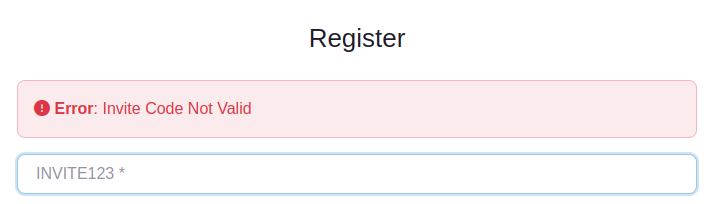